App Actions
registering additional rule actions for the rule builder
One of the ways apps can extend our platform is by registering additional actions that can be invoked in Rules.
These can be added to your app manifest:
{
...
"actions": [
{
// unique identifier for this action (must be snake_case)
"id": "notify_channel",
// human-readable description of this action
"name": "Notify Channel",
// the url in your app that will be called (POST) to execute this action
// note: as other urls, this can be relative to the manifest url
"url": "actions/notify_channel",
// optional, url to call (POST) for settings to show in the rule builder
"settings_url": "actions/notify_channel/settings",
// optional, instead of the above, when settings are fixed (independent of user)
"settings": {
"select_field_example": {
"type": "select",
"placeholder": "please select a value",
"hint": "",
"label": "",
"required": "true|false default to true",
"width": "sm|md|lg|xl",
"options": [
{
"key": "it",
"value": "IT",
"cascade": {
"it_subtypes": {
"type": "select",
"placeholder": "select a sub type",
"hint": "",
"label": "",
"required": "",
"options": [
]
}
}
},
{
"key": "sec-ops",
"value": "SecOps"
}],
"error": {
"message": "error message"
}
},
"text_field_example": {
"type": "text_input",
"label": "",
"placeholder": "",
"hint": "",
"required": "true|false"
}
}
}
]
}
For users that installed your app, these actions are shown in the Rule Builder, as additional options in the rule steps.
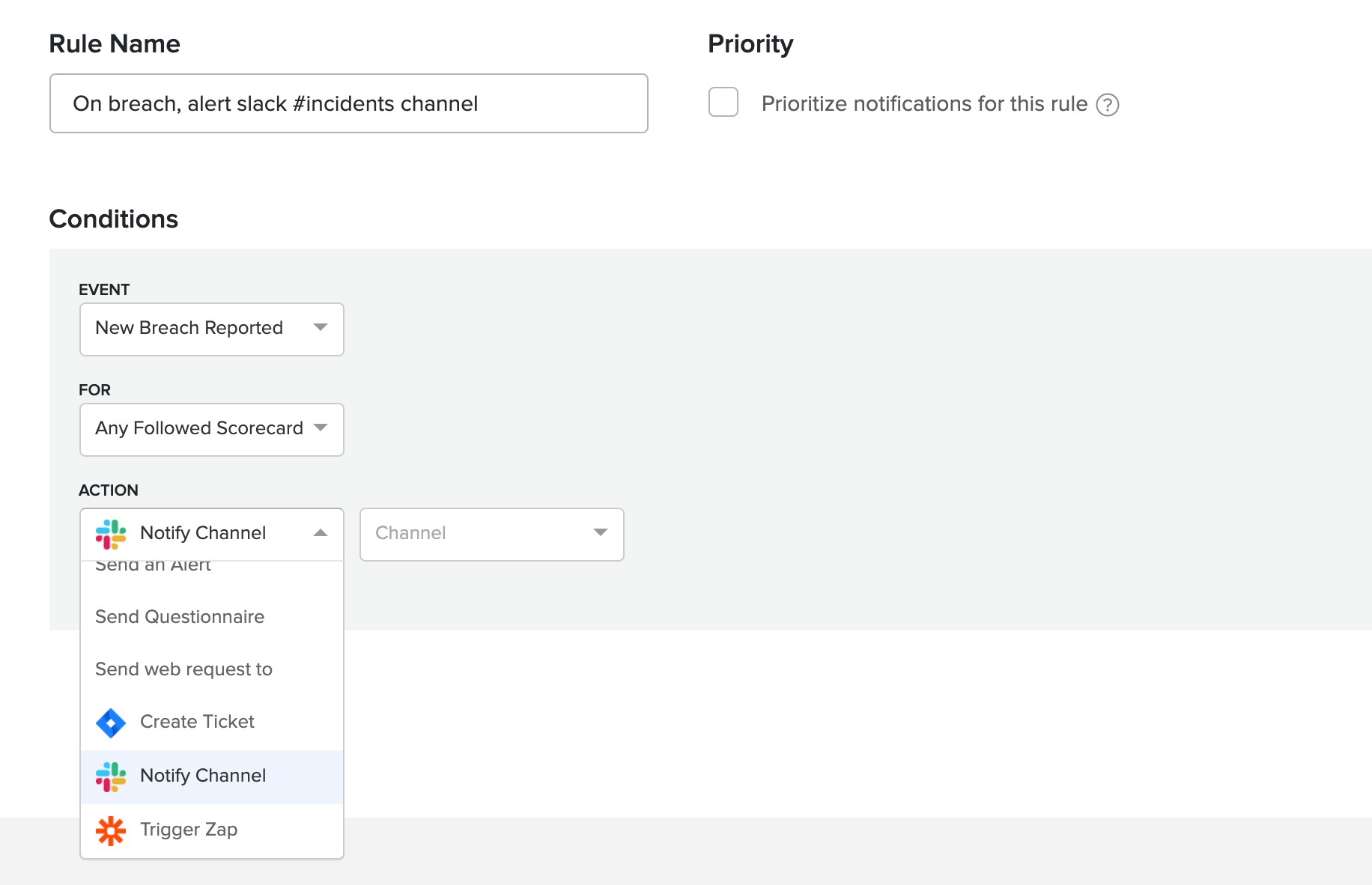
Action Settings
Sometimes an action can offer the user settings that define the action behavior. For example, when choosing the "Notify Channel" action in our Slack app, the user is shown a dropdown to select a slack channel from their slack workspace.
This is done by specifying action settings. Settings can be fixed as settings
or dynamic by specifying an endpoint in your action as settings_url
to determine it during Rule builder edit time.
When settings_url
is used, the endpoint will be called with a POST, and including any secrets in an x-ssc-app-secrets
http header, allowing you fetch options that require a form of authentication (as the slack channels in the example).
If you settings include a field with options
, a dropdown will be shown to the user:
{
"field1": {
"placeholder": "Field Name",
"options": [{
"key": "key-a",
"value": "A"
}, {
"key": "key-b",
"value": "B"
}]
}
}
Currently only dropdown fields are supported, but based on your feedback, additional field types will be added in the future.
Selected setting values will be included when executing the action.
Action Execution
When a Rule workflow is executing, your action might be invoked by sending a POST to the specified url
, including a JSON payload like this:
{
// optional, included if your action has settings
"settings": {
"parameters": {
"field1": {
"value": "A"
}
}
},
"context": {
"event": {
"type": "scorecard.changed",
"payload": {
// event details, will vary depending on the event type
}
},
"trigger": {
// describes what triggered this rule
},
"subscriptionId": "typically a rule id",
"workflow": {
"name": "rule name"
}
}
}
Simulation
Authentication
If during installation, your app sent any secrets, this will be included in an http header called x-ssc-app-secrets
, encoded as a JSON object. This header is included both when executing your action, as when calling the settings url (if any).
Requirements & Limitations for your server
- use https protocol
- accept an http POST with a json payload
- respond within 5 seconds
- respond with a 2xx status
- no response body, or a response body smaller than 100KB
Errors and Retry Support
On network errors or an http status 5xx, we'll perform a series of retries every 6hs for up to 36hs.
Note: we might limit this further depending on the responsiveness of your app.
Conditionally stopping the Rule workflow
You can optionally implement custom logic in your action to conditionally stop the execution of the Rule workflow by including in the JSON response a workflowExit
property as in the example below:
{
"workflowExit": true
}
next steps will be skipped. So this is ideal to implement steps like "Only continue if ..."
Updated about 3 years ago